Flux In-depth
The biggest problem with Meteor templates is that they need to get their data from somewhere. Unfortunately there is no good pattern provided by the core team, so everyone has to come up with custom solutions. space:flux
integrates with blaze-components, by providing a simple API to access reactive application state inside your components (provided by the stores).
Components hand over state to their managed templates, interpret (dumb) template
events and publish business actions. The stores listen to published actions and update their internal state according to its logic. The changes are reactively pushed back to the components that declared their dependency on stores by accessing their data
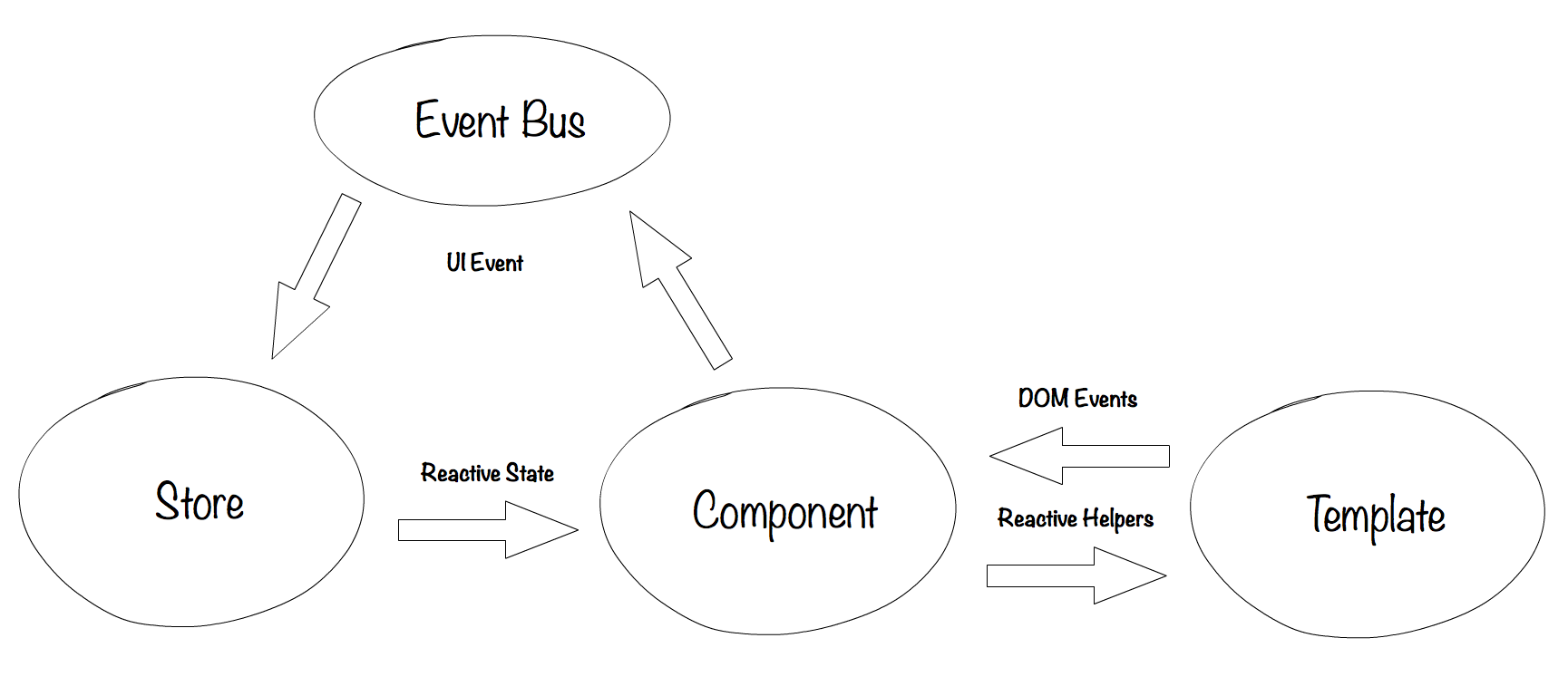
Data source is reactive
Just define a method
Space.flux.Store.extend(Todos, 'TodosStore', {
…
activeTodos() {
return this.todos.find({ isCompleted: true });
}
…
});
Data source is non-reactive, but can be inferred from other state
Add the function to the reactiveVars
return array
Reactive vars should be used for values that can be inferred by state like the current route or computed data. These won't "survive" hot-code pushes but will be rebuilt on every reload.
Example
Looking at the current route can determine the value, so after a hot-code push the value is restored.
Space.flux.Store.extend(TodoMVC, 'TodosStore', {
reactiveVars: function() {
return [{
activeFilter: this.FILTERS.ALL,
}];
}
});
This will generate an activeFilter
method on your store which can be used to get and set the reactive var.
Data source is non-reactive, and cannot be inferred from other state
Add the function to the 'sessionVars' return array
Session vars should be used for values that cannot be inferred by routes or loaded data and should survive hot-code pushes.
Example
Imagine a comment textarea where the user writes something. This should survive hot-code pushes and save the value!
Space.flux.Store.extend(TodoMVC, 'TodosStore', {
sessionVars: function() {
return [{
editingTodoId: null
}];
}
});
Updated less than a minute ago